Diving into a sea of data without a compass can be overwhelming. That’s where the elegance of simplicity comes into play, especially when it’s time to filter a table with JavaScript.
It transforms a wild tangle of information into a navigable stream, leading straight to the insights sought.
In the midst of the digital cosmos, the ability to sift through content with precision becomes a lighthouse for knowledge seekers.
Understanding how to implement and wield this powerful feature is not just a skill but a necessity for anyone looking to enhance their web creations.
This article is a journey through the art of refining table data with JavaScript—from the basic setup to implementing search inputs and onto more nuanced techniques.
Discover how to improve the user experience with thoughtful interface feedback, while ensuring every interaction is accessible and efficient. By the end, the ability to tailor data views at the click of a button will be yours.
Table of Contents
Setting Up the HTML Table for Filtering
Basic HTML Table Structure
Imagine a skeleton that holds everything up, where every bone has its right place. That’s what designing a good table feels like. You start with <table>
, and it’s like laying down the bones.
Then comes <thead>
, the skull, holding the brains—the column headers that’ll guide users. Nestled snugly below is <tbody>
, the ribs, sheltering all the data rows, each one a breath of information.
Creating this structure is not just for show; it’s about communicating clearly with all visitors. You see, semantic HTML isn’t a flashy trend—it’s the backbone of web accessibility.
Use <th>
for headers, and <tr>
for rows to tell screen readers what’s what. It’s like adding labels in a storeroom; everything gets easier to find.
Each <td>
cradles data like treasures waiting to be discovered.
Preparing the Data for the Table
Imagine you’re a chef. You’ve got ingredients—some come from a can (static), some are fresh from the market (dynamic). Your table’s taste depends on it.
Now, when dealing with static data, it’s like a recipe you know by heart. You slap it down, and it’s good to go. But with dynamic data, there’s a bit of magic involved; you pull data out of thin air—or the internet, to be precise.
Fetching data with JavaScript is like going fishing with a high-tech net—you throw in AJAX or the sleeker Fetch API, and haul in your catch.
Every pull brings in fresh data, right onto the visitors’ plates. It’s real-time, it’s lively, and oh, it makes your table show not just what’s for dinner, but what could be, if the diner so wishes.
With AJAX, you’re the old sea dog, casting wide, tried-and-tested nets.
Fetch API? That’s the spinner reel—modern, easy to handle, and oh-so-smooth. You make a call, and the data streams in, ready to be served in neat, organized rows that say, “Take your pick, it’s all delish!”
Implementing Basic Search and Filter Functionality
Creating the Search Input
See the Pen
Search Filterable Table – jQuery by Mahmudul H. Rabby (@mahmudulhrabby)
on CodePen.
Let’s dive into the ocean of data on a quest to find that shiny pearl—a specific piece of information.
The gateway to that treasure is a simple but mighty tool: the search box, an HTML input element. Picture it as a friendly sea creature, always there to help navigate through the vast waters.
Crafting this search box, it’s got to feel right, like the first dip into warm summer waters.
It begins with a <label>
, whispering hints to the users, ensuring no one’s lost at sea. Then comes the <input>
tag itself, a raft floating atop our sea of data, waiting for a keyword to paddle through the waves of information.
And let’s not forget the styling, the colors and shapes that make any exploratory journey a joy.
With CSS’s gentle waves, the search box can stand out or blend in, as bold or as subtle as a seashell on the shore. The goal is comfort; a space that beckons, “Type here, and let’s surf the data together.”
Writing the JavaScript Filter Function
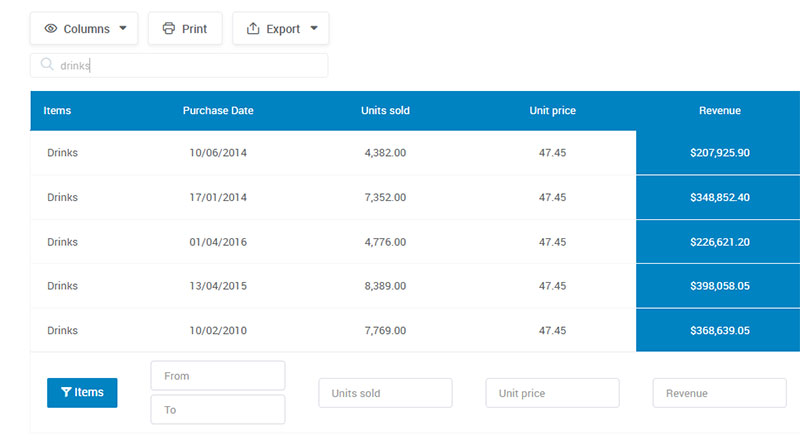
Now the adventure begins. With every keystroke in our search box, the JavaScript filter function kicks into action, like a school of dolphins weaving through the water, eager and swift, skimming the surface for hints of what lies beneath.
Hooking up to the rhythmic dance of the KeyUp event listener, our function listens intently, catching every ripple of input—every dot and dash typed by the user. As each character falls like a drop into our ocean, the script awakens, filtering through the HTML table with grace.
Much like comparing grains of sand, our function looks at each row, each table data cell, and questions: “Do you match the pattern traced in the sand by our user’s journey?”
It’s here that real-time filtering comes alive, a display of matching rows responding to the user’s call, hiding others into the depths of the ocean, leaving only the relevant ones to bask in the sunlight above.
Advanced Filtering Techniques
Column-Specific Filtering
Picture this: shelves packed with jars, each labeled with spices. Now, someone wants just the cinnamon. Wouldn’t it be nice if they could zero in on just the cinnamon shelf?
That’s what column-specific filtering feels like. It means not getting swamped by everything in the table.
Only the columns visitors care about, nothing else. They want the ‘who,’ not the ‘where’ or ‘when’? No problem. Give them a magnifying glass for names, and blur out the rest.
How is this wizardry done? With dropdowns, like little trapdoors under each column header. Click, and whoosh—you’ve got a list, pick and choose to tailor the view.
Each selection is a gateway to a cleaner look; the clutter fades, and clarity takes over, leaving a spotlight on the chosen column. Dive into the sea of data, but bring along your snorkel, focusing only on the treasures you want.
Multiple Criteria Filtering
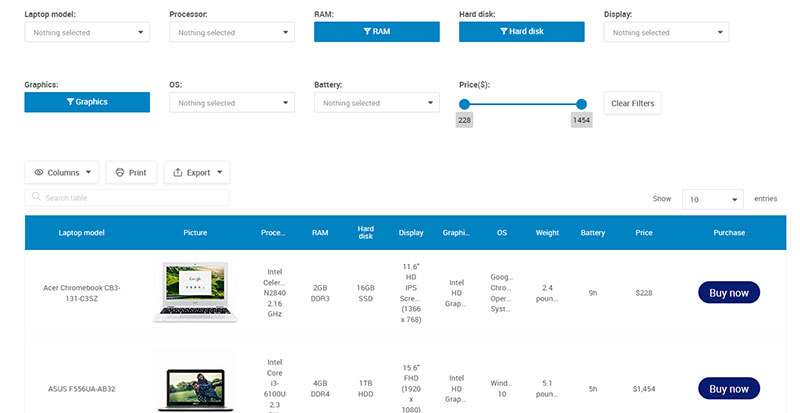
Now, here’s where it gets spicy: mixing seasonings to get that perfect flavor. Some folks want cinnamon and nutmeg.
How do we do that? We layer filters, sieves nestled within sieves, each catching finer grains, letting visitors blend their experience.
We’re talking about a confluence of filters, working in harmony. Blend the ‘who’ with the ‘what’ and maybe a dash of ‘how much’.
It’s a dance of conditions, a symphony played out on the DOM (Document Object Model), each note resonating with a user’s desire to refine their search.
And the strategies for sorting out these complex recipes of queries? Think of it as being a DJ, mixing tracks so smoothly that the transitions are felt but not heard.
JavaScript, our turntable, allows for elegant methods that spin through data effortlessly—there’s Array.prototype.filter(), trim, sort, the legendary event handling, and the sidekick, pagination.
Everything comes together in this cosmopolitan jamboree where each filter adds a stanza, a line, a verse, until the full story unfolds—coherent, crisp, and utterly customer-centric.
It resonates with the precision of a clockmaker, slicing time into perfect increments, segmenting data under multiple lenses until the view is exactly as desired.
Enhancing Filter Functionality
Debouncing for Performance Optimization
Imagine chatting away with an old friend. You don’t fire off every thought as it comes. No, you pause, you mull over things, then let your words flow. That pause? It’s like debouncing in the digital world.
See, when users type away in a search box, eager as hummingbirds, every letter sends a signal. Without debouncing, that’s a lot of asking, “Hey, got anything for me?” It’s courteous but exhausting.
So, introduce a small wait time. Each keystroke winds up a tiny timer, and it’s only when the ticking stops that we send off the request. This pause, it’s just a breath, long enough to let the system catch up but brief enough to keep it feeling snappy.
How does this sorcery work? Wrap up the function that listens to the user’s input with JavaScript’s gentle embrace.
It watches, waits for a break in the typing storm, then calls to the sea of data, “This one, this query, please.”
This isn’t just for the system’s sake; it’s a kindness to servers and a whisper to the wild web. It saves unnecessary work, like sending letters you never meant to post.
Debouncing, then, is about efficiency, a smooth operator in a world that often doesn’t know when to pause.
User Interface Feedback
Now, we get to the part that’s all about the feels—the user interface feedback. It’s the smiling waiter, the nod, the “just a moment” when you order your coffee.
Users need to know they’ve been heard, that the gears are turning.
Start with indicating active filters. Like flags on a map, they say, “You are here,” a gentle reminder of the chosen path.
This could be bolded text, a brighter color, maybe even a chip with an ‘x’ ready to dismiss the filter like a polite “no, thank you” to an offer.
And if the winds don’t blow favorably, if the nets come up empty, then what? Users need to know that too.
A message, as clear as a bell on a quiet street. Offer suggestions, “Did you mean…?” or “Try broadening your search.” It’s not about a dead end; it’s about exploring new roads.
Best Practices and Common Pitfalls
Accessibility Considerations
Imagine a world where every door is open wide, and every pathway is clear—feels good, right?
That’s the vision when we weave accessibility into the fabric of a web page. It’s about crafting a digital space that welcomes everyone, no matter how they interact with the world.
When adding features to filter a table with JavaScript, it’s not just about making it work; it’s about making it work for everyone.
It starts with ARIA—no, not a song, but almost as powerful. ARIA stands for Accessible Rich Internet Applications. It’s like a secret code that speaks directly to screen readers, telling them a story about what’s on the screen.
With ARIA roles and properties, it’s a nudge to say, “Here’s a button,” or “This is a dropdown,” guiding users with invisible cues that shout kindness and respect.
Using these tools means someone tapping their way across a keyboard or listening for cues can still dive into data with the rest of them, flying through filters and surfing rows with the same ease.
It’s about making sure the concert of interaction plays a melody that resonates with every ear, with every heart.
Performance Optimization
Now, let’s chat about the engine that powers all these fancy bits—the code that makes the magic happen. But here’s the thing: magic can be heavy. It can be like pouring molasses in winter, slow and sticky. The goal? Keep it quick, keep it nimble, like a dancer mid-pirouette.
Optimization is the fancy word, but it’s really just about being considerate—thinking about how every piece fits together, how every script runs, so that the whole machine purrs like a well-fed cat.
Start by looking at reflows and repaints—tech speak for “How often does the browser have to redraw the page?” Every tweak, every nudge to the layout is like asking the browser to repaint a masterpiece—time-consuming and, honestly, a bit of a drag.
So, cut down on the work. Be smart. If styles change, batch them together. If scripts reorder the furniture, make sure they’re not running wild like puppies on a sugar rush.
This way, when a user flicks a filter, the performance doesn’t take a nosedive—it soars, smooth and effortless.
Then, there’s efficient event handling. Like a librarian who knows exactly where every book is, every event listener should know its place—no unnecessary chatter, just swift, relevant responses.
Your beautiful data deserves to be online
wpDataTables can make it that way. There’s a good reason why it’s the #1 WordPress plugin for creating responsive tables and charts.
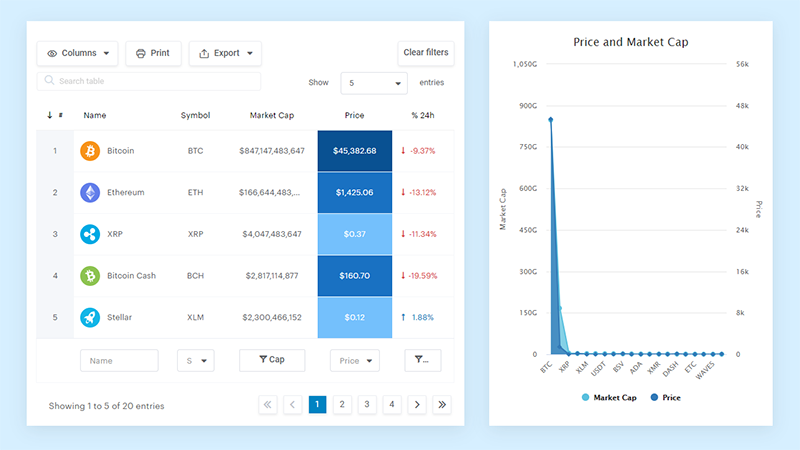
And it’s really easy to do something like this:
- You provide the table data
- Configure and customize it
- Publish it in a post or page
And it’s not just pretty, but also practical. You can make large tables with up to millions of rows, or you can use advanced filters and search, or you can go wild and make it editable.
“Yeah, but I just like Excel too much and there’s nothing like that on websites”. Yeah, there is. You can use conditional formatting like in Excel or Google Sheets.
Did I tell you you can create charts too with your data? And that’s only a small part. There are lots of other features for you.
FAQ On Filter A Table With JavaScript
How do you filter a table with JavaScript?
It begins with JavaScript listening, poised for user input.
Craft a function, hook it onto an event—usually key up—that sweeps through table rows, clasping or releasing them based on matches. Think of it as teaching the webpage a game of hide and seek with data.
Can JavaScript filter handle multiple conditions?
Absolutely, it’s like a multi-talented artist. Within the JavaScript filter function, you can set up several criteria—strings, numbers, dates—and it deftly juggles them all.
It checks each piece of data against your conditions, much like a chef tasting multiple ingredients for the perfect balance.
What are dynamic HTML tables?
Dynamic HTML tables are alive; they breathe and change. Fed by real-time data, they respond and morph with user interactions.
Populate them using AJAX or Fetch API, and they’ll display fresh data as if by magic, no page refreshes—just smooth content shifts as the user engages with the page.
How does searching in a table column work?
Column searching is like tuning into a frequency. You tell JavaScript to focus on whispers from a particular column, to ignore the noise from the rest.
Implement it by assigning search fields to each header, filtering based on specific column values with surgical precision.
Is it possible to sort and filter a table simultaneously?
Dance and sing at the same time? Why not! Sorting and filtering are two different steps that, when choreographed well, can beautifully coexist.
Sort your data, then apply filters—or vice versa—creating an environment where harmony reigns and users find their rhythm quickly.
What’s needed to implement pagination with a filtered table?
Pagination is like laying out breadcrumbs through your data. It’s a way to chunk the adventure, to not overwhelm with too much at once.
Implement it by determining a set number of rows per page, then recalculating which data to display as filters are applied or pages are turned.
How do you ensure filtering is accessible?
Whisper cues through the ARIA language—lay out landmarks with roles and properties so screen readers can narrate the journey.
Intuitive keyboard navigation is like holding a user’s hand as they cross the street, ensuring each step in the filtering process is announced and understood.
Can filtering be visually indicated in the interface?
Of course, and it should! Light up active filters like fireflies on a warm summer evening—a visual symphony that tells users where they’ve been and what they’ve set. Illuminate paths taken, softly dim other options, guiding with care, as if through a gentle, well-known trail.
Why is debouncing important in search filtering?
Imagine shouting in a crowded room. Now, imagine waiting for a pause before speaking. That’s debouncing.
It holds back the cascade of data requests until a breath has passed, streamlining communication between the user’s intent and the system’s response, preventing the dreaded freeze where thoughts and actions collide.
What are common mistakes when filtering tables?
Overcomplication is the siren song that leads to choppy waters. Keep it simple, clear, and direct, avoiding tangled webs of code.
Neglecting accessibility or performance optimization can strand users on a deserted island of frustration. Guide each interaction with care, ensuring the way back is always easy to find.
Conclusion
In threading through the intricacies of information, the art to filter a table with JavaScript morphs into a dance—a dance of clarity amidst the cacophony of data. The tableau we’ve painted together here goes beyond mere functionality; it’s a tapestry woven with the threads of accessibility, performance, and user engagement.
To cap this tale, remember, filtering is not just about narrowing down but about opening up possibilities. It’s about offering users the compass to navigate the vast seas of information effortlessly. The techniques outlined are your sails and rudder, steering through the waves of web development with purpose.
As the curtain falls on our odyssey, take these final whispers to heart:
- Keep your code as crisp as autumn air.
- Offer the user interface as an inviting path, not a labyrinth.
- And let every interaction hum a tune of efficiency and inclusivity.
With these in hand, the journey to master and implement dynamic data-filtering becomes not just attainable but a voyage worth cherishing.