Ever found yourself drowning in a sea of unsorted data within an HTML table? Using JavaScript for sorting tables not only tidies up your data presentation but also enhances user interaction and performance; a true game-changer in any web developer’s toolkit.
This crucial skill elevates table manipulation, ensuring your web applications are not only functional but also quick and responsive.
In this in-depth guide, we will venture through the nuts and bolts of implementing client-side sorting using JavaScript.
From basic sort functions and manipulating the DOM to more advanced techniques like pagination and multi-column sorting, you’ll learn how to make your tables supremely user-friendly and efficient.
By the end, you’ll not only be equipped to implement these features but will also understand how to optimize your sorting for performance and user experience, ensuring your tables work seamlessly across different browsers and devices.
Table of Contents
- Fundamentals of HTML Table Structure
- Making a Simple Sortable Table
- Advanced Sorting Techniques
- Enhancing the User Interface
- Pagination
- Performance Optimization
Table of Contents
Fundamentals of HTML Table Structure
Basic HTML Table Markup
If you’re diving into HTML table creation, understanding the fundamental markup is essential. Let’s talk elements: the classic table, thead, tbody, tr, th, and td. Each one plays a unique role in structuring your data, giving it both form and function.
Table, Thead, Tbody, Tr, Th, Td Elements
Imagine you’re setting up a dinner party. The table is your big dining area. Inside, you have sections: the thead for the header, kind of like your nameplates and fancy centerpieces, and tbody for the main content where your dishes go.
- tr stands for table row, each like a single row of dishes.
- th, table header, these are your labeled headers, maybe the names on your place cards.
- td, table data, where each dish or piece of cutlery sits, effectively the meat and potatoes of your setup.
<table>
<thead>
<tr>
<th>Header 1</th>
<th>Header 2</th>
</tr>
</thead>
<tbody>
<tr>
<td>Data 1</td>
<td>Data 2</td>
</tr>
</tbody>
</table>
Boom! Your basic structure is set.
Example of a Simple HTML Table
A quick practical example? Sure, let’s assume we’re organizing a schedule of your front-end development tasks.
<table>
<thead>
<tr>
<th>Task</th>
<th>Deadline</th>
</tr>
</thead>
<tbody>
<tr>
<td>Design HTML Tables</td>
<td>April 1</td>
</tr>
<tr>
<td>JavaScript Sorting Implementation</td>
<td>April 5</td>
</tr>
</tbody>
</table>
Clear, readable, and easy to follow.
Adding IDs and Classes for Targeting
Dressing up your table with IDs and classes isn’t about vanity—it’s about precision and flexibility in your styling and scripting.
Importance of IDs and Classes
Why bother with IDs and classes? Think of them as your table’s backstage passes. IDs are unique; they get you into exclusive areas—use them for single, specific elements. Classes, on the other hand, are more like VIP badges; they grant access to a group of elements that share the same style or behavior.
IDs make elements unique targets in a crowd, critical for pinpoint actions. Classes ensure uniform styling or behavior across similar elements.
Examples
Let’s add some flair:
<table id="devSchedule" class="task-table">
<thead class="table-head">
<tr>
<th>Task</th>
<th>Deadline</th>
</tr>
</thead>
<tbody class="table-body">
<tr class="completed">
<td>Design HTML Tables</td>
<td>April 1</td>
</tr>
<tr id="currentTask">
<td>JavaScript Sorting Implementation</td>
<td>April 5</td>
</tr>
</tbody>
</table>
Notice how IDs and classes articulate different needs? IDs for individual elements like #currentTask
, and classes for groups like .task-table
and .table-body
.
Enchanting tables with flexibility for both CSS and JavaScript tweaking. This paves the way for interactive magic, like sorting tables with JavaScript, which can be effortlessly enhanced by binding event listeners to those pristine IDs and classes.
The structure breathes, setting the stage for dynamic content and sorting mechanisms. An elegant ensemble where each element knows its role, every class and ID ready to step into the limelight when invoked by the script or style sheet. The concert of tables begins here, with structure and precision laying down the melody.
Making a Simple Sortable Table
Setting Up the HTML Markup
The magic begins with your table structure. Yes, the skeletal frame that underpins the entire sortable mechanism. Get this wrong, and you might as well be rowing a boat with a toothpick. Here’s how to set it up so everything locks into place naturally.
Preparing the Table Structure
First, we need our table to shine. Craft it with meticulous precision, ensuring every element knows its part. Each header, each row has got to align perfectly—almost like a well-choreographed dance.
<table id="sortableTable">
<thead>
<tr>
<th>Item</th>
<th>Price</th>
<th>Quantity</th>
</tr>
</thead>
<tbody>
<tr>
<td>Apples</td>
<td>2.50</td>
<td>5</td>
</tr>
<tr>
<td>Bananas</td>
<td>1.50</td>
<td>10</td>
</tr>
<tr>
<td>Oranges</td>
<td>3.00</td>
<td>8</td>
</tr>
</tbody>
</table>
Everything aligns like stars in a coder’s sky, each tag wrapping your data lovingly.
Adding Necessary Attributes
Attributes—these tiny tinker bells—sprinkle magic into your table. You need to subtly add IDs to the headers to make them into sortable titans. They’re the switches to your JavaScript magic.
<th id="itemHeader">Item</th>
<th id="priceHeader">Price</th>
<th id="quantityHeader">Quantity</th>
IDs turn simple table headers into powerful, sortable columns just waiting for that JavaScript touch.
Basic JavaScript for Sorting
Once the canvas is set, it’s time to paint with JavaScript. We’re talking about functions that breathe life into your table, transforming it from static data to interactive brilliance.
JavaScript Functions for Sorting
Let’s whip up a function, a sorcerer of sorts, that will handle the sorting.
function sortTable(columnIndex) {
var table = document.getElementById("sortableTable");
var rows = Array.prototype.slice.call(table.querySelectorAll("tbody > tr"));
rows.sort(function(rowA, rowB) {
var cellA = rowA.cells[columnIndex].textContent;
var cellB = rowB.cells[columnIndex].textContent;
// Handle numerical values
if (!isNaN(cellA) && !isNaN(cellB)) {
return cellA - cellB;
}
// Default to string comparison
return cellA.localeCompare(cellB);
});
rows.forEach(function(row) {
table.querySelector("tbody").appendChild(row);
});
}
This function? It’s a shape-shifting wizard, sorting any column based on index while quietly assessing if it’s dealing with numbers or text—no assumptions.
Event Listeners for Table Headers
But wait, our table isn’t fully alive until it responds to the user’s touch. Enter event listeners. With these, every header click triggers our sorting sorcery.
document.getElementById("itemHeader").addEventListener("click", function() {
sortTable(0);
});
document.getElementById("priceHeader").addEventListener("click", function() {
sortTable(1);
});
document.getElementById("quantityHeader").addEventListener("click", function() {
sortTable(2);
});
The headers now vibrate with potential energy, waiting for a single click to burst into action.
Example Code and Explanation
Let’s pull it all together, paint the full picture. This is the art of making pure magic with code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Sortable Table</title>
<script>
function sortTable(columnIndex) {
var table = document.getElementById("sortableTable");
var rows = Array.prototype.slice.call(table.querySelectorAll("tbody > tr"));
rows.sort(function(rowA, rowB) {
var cellA = rowA.cells[columnIndex].textContent;
var cellB = rowB.cells[columnIndex].textContent;
if (!isNaN(cellA) && !isNaN(cellB)) {
return cellA - cellB;
}
return cellA.localeCompare(cellB);
});
rows.forEach(function(row) {
table.querySelector("tbody").appendChild(row);
});
}
document.addEventListener("DOMContentLoaded", function() {
document.getElementById("itemHeader").addEventListener("click", function() {
sortTable(0);
});
document.getElementById("priceHeader").addEventListener("click", function() {
sortTable(1);
});
document.getElementById("quantityHeader").addEventListener("click", function() {
sortTable(2);
});
});
</script>
</head>
<body>
<table id="sortableTable">
<thead>
<tr>
<th id="itemHeader">Item</th>
<th id="priceHeader">Price</th>
<th id="quantityHeader">Quantity</th>
</tr>
</thead>
<tbody>
<tr>
<td>Apples</td>
<td>2.50</td>
<td>5</td>
</tr>
<tr>
<td>Bananas</td>
<td>1.50</td>
<td>10</td>
</tr>
<tr>
<td>Oranges</td>
<td>3.00</td>
<td>8</td>
</tr>
</tbody>
</table>
</body>
</html>
Look at it—an HTML table framework enhanced by IDs, paired with a JavaScript engine that sorts columns based on pure user interaction.
For those practicing sorting tables with JavaScript, this example embodies an elegant blend of structure and dynamism, laying down a baseline for more complex table interactions.
Do you want a simpler way? Just use wpDataTables
wpDataTables can help you generate sortable tables without writing any code. There’s a good reason why it’s the #1 WordPress plugin for creating responsive tables and charts.
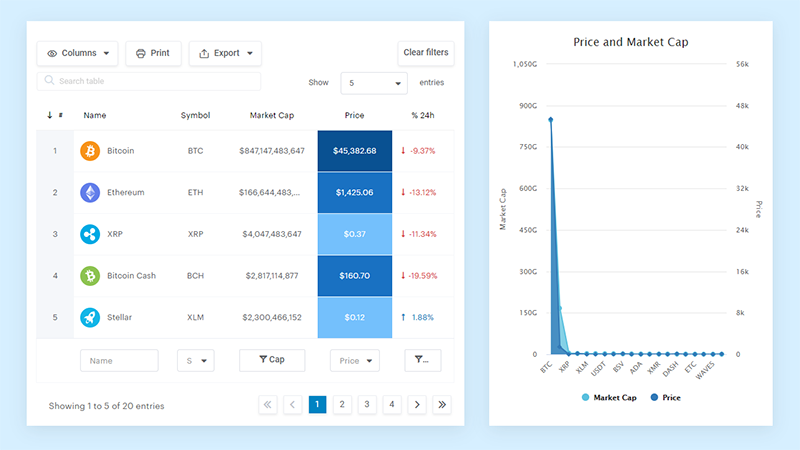
And it’s really easy to do something like this:
- You provide the table data
- Configure and customize it
- Publish it in a post or page
And it’s not just pretty, but also practical. You can make large tables with up to millions of rows, or you can use advanced filters and search, or you can go wild and make it editable.
“Yeah, but I just like Excel too much and there’s nothing like that on websites”. Yeah, there is. You can use conditional formatting like in Excel or Google Sheets.
Did I tell you you can create charts too with your data? And that’s only a small part. There are lots of other features for you.
Advanced Sorting Techniques
Multi-Column Sorting
Step up your game. Sorting by a single column? It’s basic. Real power comes through sorting multiple columns.
Sorting by Multiple Columns
Imagine a sophisticated dinner party. You’re sorting guests first by age, then by favorite wine. The clever sorting mechanism isn’t deterred by complexity. For multi-column sorting, we toy with layers of criteria.
function multiColumnSort(rows, columns) {
return rows.sort(function(rowA, rowB) {
for (let column of columns) {
let cellA = rowA.cells[column.index].textContent;
let cellB = rowB.cells[column.index].textContent;
if (cellA !== cellB) {
if (!isNaN(cellA) && !isNaN(cellB)) {
return column.order * (cellA - cellB);
}
return column.order * cellA.localeCompare(cellB);
}
}
return 0;
});
}
That snippet? It’s a sleek operator, sorting based on an array of column objects where each has an index and order. Ascend or descend—for it, it matters not.
Implementing Multi-Column Sort in JavaScript
Combine this elegant function with your table structure. Imagine you have several criteria. Reshuffle those table rows seamlessly, quietly marveling at the power of JavaScript.
var rows = Array.from(document.querySelector("tbody").rows);
var columns = [{index: 0, order: 1}, {index: 1, order: -1}]; // Example: Sort first column ASC, second DESC
var sortedRows = multiColumnSort(rows, columns);
sortedRows.forEach(row => document.querySelector("tbody").appendChild(row));
Elegance in motion.
Data Types Handling
Sorting is a nuanced art. Numbers, dates, text—each demands a deft touch. Different data types, different strokes.
Sorting Numerical Values
Numerical values are straightforward; they crave mathematical comparisons.
function sortNumerical(a, b) {
return a - b;
}
Clean and direct. Your sorted output aligns perfectly.
Sorting Dates and Other Data Types
Dates, however? They’re finicky. They need conversion, that touch of parsing magic.
function sortDate(a, b) {
return new Date(a) - new Date(b);
}
Dates bow to their new order. And beyond that, custom data types — all designed to harmonize under well-crafted sort functions.
Numbers, text, dates—all dancing to the same JavaScript tune, embodying both power and simplicity. Herein lies the real art of sorting tables with JavaScript, an interplay of elements and code, creating dynamic, interactive tables that respond seamlessly to user actions.
Marvel at the transformation—from static to dynamic, living data tables on a digital canvas.
Enhancing the User Interface
Adding Sort Indicators
Tables and indicators—like thunder and lightning. Visual cues are everything.
Visual Cues for Sorted Columns
Imagine a cliffhanger without an exclamation point. Boring, right? Your users need to know which column just changed their world. Enter sort indicators.
Adding an arrow? Child’s play. Keeping it dynamic? That’s the trick.
function setSortIndicator(columnHeader, direction) {
const indicator = direction === 'asc' ? '▲' : '▼';
clearIndicators(); // A function to clear previous indicators
columnHeader.textContent += ' ' + indicator;
}
Give your headers that flair. Instantly, users know which column is now the protagonist.
Changing Indicator on Click
Dynamic headers? They’re a must. You click, it changes—like magic. First an empty indicator, a blank canvas. Then a click breathes life into it, turning simplicity into interaction.
document.querySelectorAll('th').forEach(header => {
header.addEventListener('click', () => {
const direction = header.getAttribute('data-sort') === 'asc' ? 'desc' : 'asc';
header.setAttribute('data-sort', direction);
setSortIndicator(header, direction);
sortTable(header.cellIndex, direction);
});
});
Ah, the elegance. One click and arrows dance, directions flip, the table metamorphoses. JavaScript conducts this symphony.
Styling Sorted Tables with CSS
CSS isn’t just about aesthetics. It’s about making elements sing. Voicing their purpose without a word.
Basic Styling Techniques
Start with simplicity. Borders, padding, the subtle elegance of a shadow. Tables are canvases—the CSS, your brushstrokes.
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid #ddd;
padding: 8px;
}
th {
cursor: pointer;
background-color: #f2f2f2;
}
Every line of CSS, a delicate stroke adding layers to your table. Seamlessly turning basic HTML into a responsive, delightful interface.
Highlighting Sorted Columns
Highlighting isn’t just about visibility. It’s focus. It draws the eye, guiding users like a lighthouse through the foggy sea of data.
th.sorted-asc {
background-color: #d1e7fd;
}
th.sorted-desc {
background-color: #f4cccc;
}
Announce which piece of data just danced to the top. Users need not search—they feel the change. Sorting tables with JavaScript becomes an immersive experience.
Users float, engaged, eyes drawn exactly where you want them. Data is no longer just information; it’s a story, told dynamically, elegantly.
Pagination
Importance of Pagination for Large Data Sets
A flood of data. A deluge. Pagination is the lifeboat. Vast swaths of information made manageable. Like a novel with chapters, it breaks everything into digestible parts.
Improving Load Times and Usability
With pagination, speed is king. Your massive dataset, once a behemoth, now prints onto the screen almost instantly. Users don’t wait; they navigate fluidly, their experience seamless.
Faster load times aren’t a luxury—they’re essential. Constrain what you load, keep it light. You don’t bring the whole library when you’re looking for one book.
User Experience Considerations
Ever felt overwhelmed by a wall of text? Without pagination, tables do that. Slice it into pieces. Each page, a new beginning. Users breathe easier, their journey effortless.
Pagination isn’t just for performance. It’s user experience. By limiting visible data, you create space. Space for eyes to rest, focus to sharpen. No confusion. Only clarity.
Implementing Pagination with JavaScript
JavaScript, our ever-willing accomplice. It takes the reins, paginates the data, adds navigational charm. Welcome to the modern age of client-side scripting.
Creating Paginated Views
First, divide. Break the dataset.
function paginate(rows, itemsPerPage) {
const pages = [];
for (let i = 0; i < rows.length; i += itemsPerPage) {
pages.push(rows.slice(i, i + itemsPerPage));
}
return pages;
}
Rows form smaller groups, pages emerge. An intuitive break from the chaos.
Navigation Controls
Clicks command change. Page forward, backward. Jump to the first, leap to the last. Simple buttons, immense power.
function renderPaginationControls(totalPages) {
const container = document.querySelector('#pagination-controls');
for (let i = 1; i <= totalPages; i++) {
const button = document.createElement('button');
button.textContent = i;
button.addEventListener('click', () => updateTable(i));
container.appendChild(button);
}
}
Navigation. A few buttons, and users control the flow of data. Like turning pages in a book.
Example Code and Explanation
Connecting dots. Turning theory into tangible. Your table, paginated. Rows forming structured groups—each interaction a step forward.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Paginated Table</title>
<style>
#pagination-controls { margin-top: 10px; }
#pagination-controls button { margin-right: 5px; }
</style>
</head>
<body>
<table id="dataTable">
<thead>
<tr>
<th>Item</th>
<th>Price</th>
</tr>
</thead>
<tbody></tbody>
</table>
<div id="pagination-controls"></div>
<script>
const rows = [
['Apples', 2.50], ['Bananas', 1.50], ['Oranges', 3.00],
['Grapes', 4.00], ['Pineapple', 2.80], ['Mango', 3.50],
['Strawberries', 2.00], ['Blueberries', 3.80], ['Peaches', 2.30]
];
const itemsPerPage = 3;
const paginatedData = paginate(rows, itemsPerPage);
renderPaginationControls(paginatedData.length);
updateTable(1);
function paginate(rows, itemsPerPage) {
const pages = [];
for (let i = 0; i < rows.length; i += itemsPerPage) {
pages.push(rows.slice(i, i + itemsPerPage));
}
return pages;
}
function renderPaginationControls(totalPages) {
const container = document.querySelector('#pagination-controls');
for (let i = 1; i <= totalPages; i++) {
const button = document.createElement('button');
button.textContent = i;
button.addEventListener('click', () => updateTable(i));
container.appendChild(button);
}
}
function updateTable(page) {
const tableBody = document.querySelector('#dataTable tbody');
tableBody.innerHTML = '';
paginatedData[page - 1].forEach(row => {
const tr = document.createElement('tr');
row.forEach(cell => {
const td = document.createElement('td');
td.textContent = cell;
tr.appendChild(td);
});
tableBody.appendChild(tr);
});
}
</script>
</body>
</html>
Rows, buttons, pages. JavaScript weaving magic. Sorting tables with JavaScript becomes a multidimensional experience, not just a linear chore.
Pagination. It isn’t just a technique—it’s the bridge between endless data and user simplicity. Every click, a new chapter.
Performance Optimization
Efficient Sorting Algorithms
Sorting algorithms are like flavors of ice cream; each has its own appeal and place. Performance is key, and picking the right one can make or break your table.
Comparison of Sorting Algorithms
Bubble sort, quicksort, merge sort—each a character in the grand play of data manipulation. Want a list? Sure, but let’s make it interesting.
- Bubble Sort: Simple, quaint. It compares each pair and swaps. Easy to understand but sluggish, like an ambling stroll in the park.
- QuickSort: The sprinter. It divides and conquers, splitting arrays then stitching them back together faster than you can say “sort”. Efficient and elegant.
- Merge Sort: Think of it as a methodical librarian. It divides arrays into smaller parts, sorts them, then merges back. Stable, reliable, but can be overkill for small datasets.
Choose wisely. Performance varies by context—small datasets may not need a lightning-fast algorithm, but large sets? Speed is the essence.
Choosing the Right Algorithm
What’s your table’s nature? The data’s demeanor? No single solution for all. Here, context is king.
function quickSort(arr) {
if (arr.length <= 1) return arr;
let pivot = arr[Math.floor(arr.length / 2)];
let less = arr.filter(i => i < pivot);
let equal = arr.filter(i => i === pivot);
let greater = arr.filter(i => i > pivot);
return quickSort(less).concat(equal, quickSort(greater));
}
QuickSort: the racehorse. Fast, powerful, splitting data with grace.
Minimizing Reflows and Repaints
Ever felt the lag when scrolling a website? That’s reflow and repaint: behind-the-scenes chaos in rendering. Reduce them for snappier UX, less frustration.
Understanding Reflows and Repaints
A reflow? It’s like rearranging your living room. Move one thing and everything shifts to follow. A repaint? It’s changing the wall color—visual changes but no structure shift.
Reflow happens when you alter the layout, triggering the browser to calculate new positions.
Repaint? When you change aesthetics like colors or visibility. Less intensive, but still costly.
Techniques to Minimize Performance Issues
Efficiency isn’t just about speed; it’s reducing unnecessary effort. How?
- Batch Style Changes: Group CSS changes. Fewer interruptions, smoother rendering.
- Class Toggles: Instead of repeatedly changing individual styles, toggle classes. Less work for the browser, better performance.
- Document Fragments: Manipulate off-screen. Apply changes, then inject into the DOM.
function updateTableFragmented(rows) {
const fragment = document.createDocumentFragment();
rows.forEach(row => fragment.appendChild(row));
document.querySelector('tbody').appendChild(fragment);
}
Here, a DocumentFragment acts as a backstage. Gather changes out of sight, then present the polished result.
Using Document Object Model (DOM) wisely—building, changing off-screen—ensures fewer reflows, quick paints. Performance optimization blends code sophistication with rendering efficiency.
Crafting responsive, performant tables? It’s not just about sorting tables with JavaScript but refining the dance between data and display. Embrace optimal algorithms, minimize reflow, and you elevate user experience.
FAQ On Using JavaWScript For Sorting Tables
How do I implement basic sorting of a table with JavaScript?
Sorting HTML tables with JavaScript starts by selecting the table element through DOM manipulation. Attach an event listener to table headers that triggers a function, sorting rows based on clicking. Utilize the JavaScript sort() method to reorder the array of table rows.
Can JavaScript sort tables by multiple columns?
Absolutely. For multi-column table sort, extend the basic sort function to consider additional columns when the primary column values are equivalent.
This involves tweaking the sorting function to prioritize primary column values and subsequently adjust to secondary ones as needed.
What is the best way to handle sorting in large tables?
For large sortable tables, consider implementing pagination or splitting the data into smaller chunks. This enhances performance by not overloading the browser.
Incorporate efficient JavaScript sorting functions and analyze performance benchmarks to ensure responsiveness.
How do I reverse the sort order using JavaScript?
Toggle sorting order in JavaScript by using a flag variable. This toggle sort order JavaScript scheme flips the sorting direction each time the user clicks the table header—typically by multiplying the comparison results by -1, effectively reversing the order.
Are there JavaScript libraries to simplify table sorting?
Indeed, numerous JavaScript libraries are designed for sorting, enhancing the functionality without extensive coding. Libraries like DataTables or SortTable provide built-in sorting, pagination, and even filtering capabilities which you can integrate quickly.
How to sort different data types in a JavaScript table?
When you’re sorting different data types in tables, ensure your sorting function is designed to handle numbers, strings, dates, etc., appropriately.
Differentiate by implementing type-specific sorting within your JavaScript function to maintain data type integrity and validation.
How does sorting impact user experience in web applications?
Properly implemented table sorting improves usability by making data interpretation straightforward.
It enhances the overall user interaction, making tables a powerful point of interaction on your web application, therefore significantly boosting the user experience.
Can sorted tables be made to work seamlessly across different browsers?
Cross-browser compatibility is crucial. Ensure your JavaScript code adheres to standards that are supported across major browsers.
Test sorting features in various environments to verify browser compatibility. This is particularly vital for maintaining functionality and appearance across platforms.
How do I make my JavaScript sorting function accessible?
To achieve accessibility in JavaScript table sorting, use proper ARIA roles and properties. Ensure that all interactive elements are accessible via keyboard and properly narrated by screen readers, encompassing a broad range of users.
What are some common mistakes to avoid when sorting tables with JavaScript?
Common pitfalls include not accounting for upper and lower case differences in text sorts, not validating data types before sorting, and poor handling of null or undefined values. Avoid these by rigorous testing and ensuring your JavaScript sorting functions are robust.
Conclusion
Diving deep into the world of using JavaScript for sorting tables has revealed a rich landscape of possibilities for enhancing web applications. Employing JavaScript sorting functions not only streamlines interaction but also significantly elevates the user’s experience by presenting data in an orderly, digestible format.
- Mastering client-side manipulation with techniques such as dynamic table sorting promises impressive performance optimization.
- Leveraging libraries for table sorting refines the process, saving time, and expanding functionalities like multi-column sorting and responsive layouts.
By incorporating these insights into your web projects, you ensure that your tables are not merely data holders but powerful tools enhancing your site’s usability and appeal. As data continues to drive decision-making, mastering this skill is not just useful—it’s essential to stand out in the digital arena.