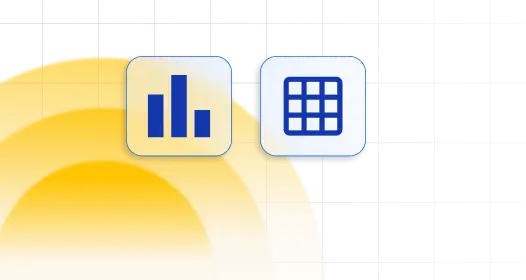
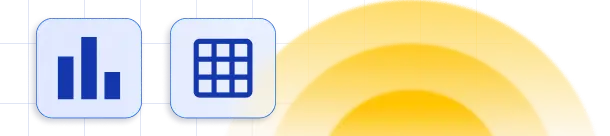
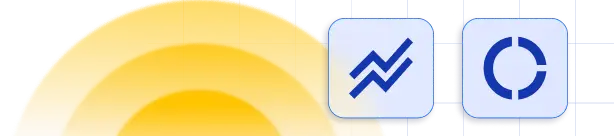
If you have an SSL certificate, and you’re having issues with tables linked to Google Spreadsheets, or if you’re creating tables linked to JSON files which require authorization, instead of modifying the code of wpDataTables (which would be overwritten with every update), you can use our hook “wpdatatables_curl_get_data”.
For JSON files, please take a look at the commented example for authentication. The custom code should be added to functions.php file of your theme, or the child theme.
function filterCURLOptions($data, $ch, $url){ // // $data (null) is set to null if there are available filter // // $ch cURL handle returned by curl_init(). // // $url (string) is URL of existing source(JSON, Google Sheet or PHP serialized array) // // Here you will insert your logic, because there are different JSON authentication // https://www.php.net/manual/en/function.curl-setopt.php // // Please note that this function is used for creating tables from JSON,Google sheets and PHP serialezed array // So you will need to filter it with some if statement based on $url parametar // // Useful links // // https://stackoverflow.com/questions/30426047/correct-way-to-set-bearer-token-with-curl // https://stackoverflow.com/questions/60183353/php-how-to-make-a-get-request-with-http-basic-authentication // https://stackoverflow.com/questions/44331346/php-api-call-with-curl-and-authentication/44332142 // https://stackoverflow.com/questions/2140419/how-do-i-make-a-request-using-http-basic-authentication-with-php-curl // Your code.... return $data; } add_filter('wpdatatables_curl_get_data','filterCURLOptions', 10, 3);
The following code is an example which is used for SSL of Google Spreadsheets:
function filterCURLOptions($data, $ch, $url){ curl_close($ch); $new_ch = curl_init(); $timeout = 5; $agent = 'Mozilla/5.0 (Windows NT 6.2; WOW64; rv:17.0) Gecko/20100101 Firefox/17.0'; curl_setopt($new_ch, CURLOPT_URL, $url); curl_setopt($new_ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($new_ch, CURLOPT_SSL_VERIFYHOST, false); curl_setopt($new_ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($new_ch, CURLOPT_CONNECTTIMEOUT, $timeout); curl_setopt($new_ch, CURLOPT_USERAGENT, $agent); curl_setopt($new_ch, CURLOPT_REFERER, site_url()); curl_setopt($new_ch, CURLOPT_FOLLOWLOCATION, true); $data = curl_exec($new_ch); if (curl_error($new_ch)) { $error = curl_error($new_ch); curl_close($new_ch); wp_die($error); } if (strpos($data, '')) { wp_die(__('wpDataTables was unable to read your Google Spreadsheet, probably it is not published correctly. You can publish it by going to File -> Publish to the web ', 'wpdatatables')); } $info = curl_getinfo($new_ch); curl_close($new_ch); if ($info['http_code'] === 404) { return NULL; } return $data; } add_filter('wpdatatables_curl_get_data','filterCURLOptions', 10, 3);
This will work for tables made from Google Spreadsheets (regular method, not tables created with Google Sheets API), JSON files and Serialized PHP Arrays.
To get your hands on wpDataTables Lite, please enter your email address below. We’ll send you a direct download link and keep you updated on existing features along with helpful tips and tricks!